React Native Development
Push Notification with Firebase Cloud Messaging in React Native
February 21, 2025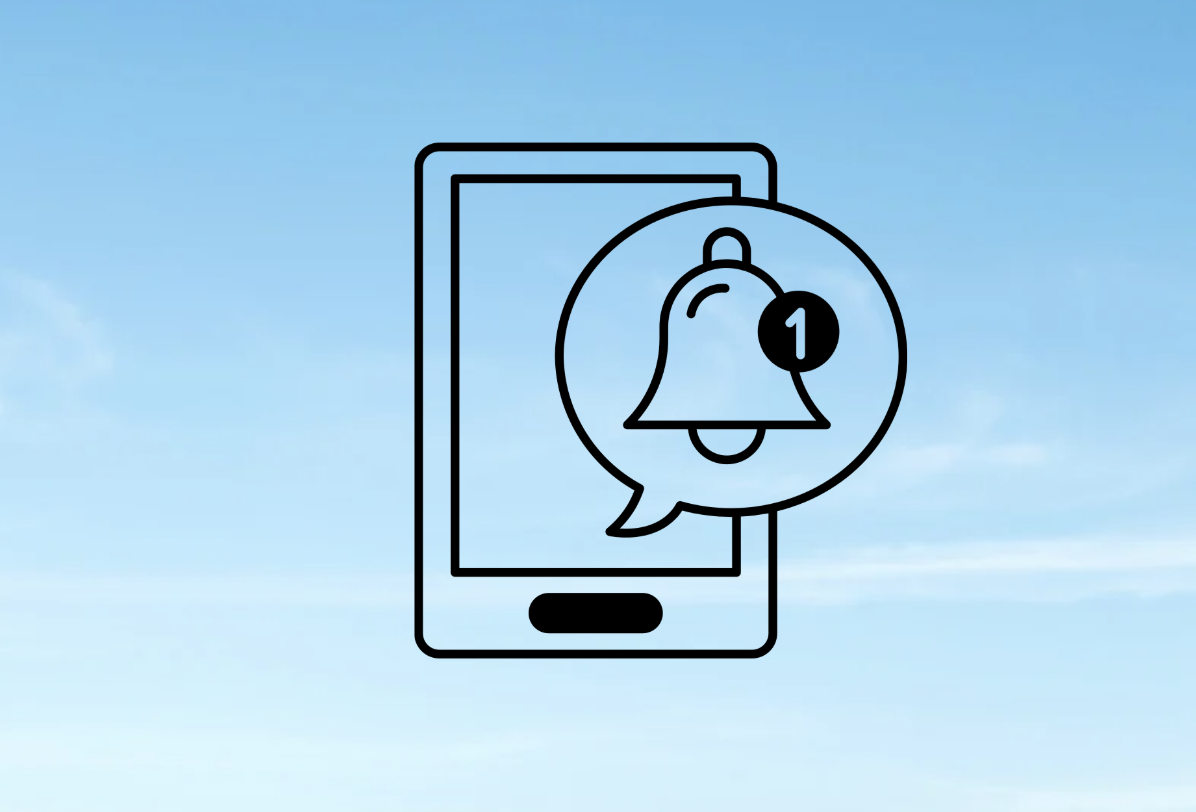
Push notifications are an essential feature of modern mobile apps, allowing businesses to engage users by sending timely updates, alerts, and offers. In React Native, Firebase Cloud Messaging (FCM) can be used for sending push notifications. With the help of react-native-firebase and Notifee, implementing this feature becomes more straightforward and powerful.
In this guide, I’ll walk you through the process of setting up Firebase Cloud Messaging within your React Native project. You’ll learn how to handle incoming push notifications, ensuring that your app can respond appropriately to messages sent from the cloud. Whether you’re sending alerts, updates, or personalized content, Firebase Cloud Messaging provides a robust solution to help you keep your users engaged and informed. Let’s dive into the world of push notifications in React Native and unlock the potential of FCM to enhance your app’s user experience.
“We must be willing to let go of the life we planned so as to have the life that is waiting for us.” — Joseph Campbell
Firebase Cloud Messaging is a cloud-based service that allows developers to send messages and notifications to users on various platforms, including iOS, Android, and web applications. By harnessing the capabilities of FCM, you can deliver personalized, timely content to your users, enhancing their overall experience with your app.
Prerequisites
Before starting, ensure you have the following:
• Basic knowledge of React Native and Firebase.
• Node.js and npm installed.
• A React Native app set up.
• Firebase project configured (if not, create one from the Firebase Console).
To accomplish what we need first we have to install some dependencies. We’ll use 3 different libraries for this.
- The library used for obtaining and managing notification permissions, react-native-permissions
- The library used for Firebase and Firebase cloud messaging integration, react-native-firebase
- The library used for receiving and displaying notifications, notifee/react-native
yarn add @react-native-firebase/app
@react-native-firebase/messaging
react-native-permissions
@notifee/react-native
Configure Firebase in ioscd ios/ && pod install
To configure Firebase for iOS, we need to:
Add Firebase iOS SDK
• Download the GoogleService-Info.plist from the Firebase console.
• Drag and drop this file into your Xcode project, ensuring the “Copy items if needed” option is checked.
Modify iOS Project Settings
1. Set Deployment Target
In Xcode, set the deployment target to 11.0 or higher. This is required for Firebase Messaging and Notifee.
2. Enable Push Notifications
• Go to Signing & Capabilities in Xcode for your target.
• Add Push Notifications under the “Capability” section.
• Add Background Modes and enable Remote notifications.
Update AppDelegate.m
Open AppDelegate.m and modify it to support Firebase and Notifee notifications:
1. Import Firebase and Notifee
At the top of AppDelegate.m, add:#import <Firebase.h>
#import <RNCPushNotificationIOS.h>
2. Configure Firebase
In the didFinishLaunchingWithOptions method, initialize Firebase:- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions {
[FIRApp configure];
return YES;
}
Configure Firebase in Android
• Add your google-services.json file (from Firebase Console) to your android/app folder.
- Modify the android/build.gradle file by adding:
classpath ‘com.google.gms:google-services:4.3.10’
- Modify the android/app/build.gradle file to apply the google-services plugin:
apply plugin: ‘com.google.gms.google-services’
Setting Up Firebase Cloud Messaging (FCM)
- Enable Firebase Messaging
- Go to the Firebase console and sign in/up.
- Add a project by clicking the “Add project” button and give a name to the project.
- Follow the steps and create a project.
- You created the project successfully. Now you’re on the overview page.
- We need to add apps to Firebase, for both Android and iOS.
- Requesting User Permissions
Now we must request notification permissions from users to send push notifications. This can be done using the messaging module.import messaging from ‘@react-native-firebase/messaging’;
async function requestUserPermission() {
const authStatus = await messaging().requestPermission();
const enabled =
authStatus === messaging.AuthorizationStatus.AUTHORIZED ||
authStatus === messaging.AuthorizationStatus.PROVISIONAL;
if (enabled) {
console.log(‘Notification permission enabled.’);
} else {
console.log(‘Notification permission not granted.’);
}
}
Call requestUserPermission() during the app initialization.
- Get the FCM Token
The device needs a unique token to receive notifications. You can retrieve this token using the following code:import messaging from ‘@react-native-firebase/messaging’;
async function getFcmToken() {
const token = await messaging().getToken();
console.log(‘FCM Token:’, token);
}
useEffect(() => {
getFcmToken();
}, []);
This token will later be used to send notifications to specific devices from Firebase.
Handling Incoming Notifications
Firebase provides three scenarios to handle notifications: while the app is in the foreground, background, or terminated state.
• Foreground Notifications
To handle notifications in the foreground, you will use Notifee, a powerful library that allows you to show customized notifications when the app is active.import messaging from ‘@react-native-firebase/messaging’;
import notifee, { AndroidImportance } from ‘@notifee/react-native’;
messaging().onMessage(async (remoteMessage) => {
console.log(‘Message received in foreground:’, remoteMessage);
await notifee.displayNotification({
title: remoteMessage.notification.title,
body: remoteMessage.notification.body,
android: {
channelId: ‘default’,
importance: AndroidImportance.HIGH,
},
});
});
Notifee allows customization of notifications, such as adding images, custom sounds, and priority settings, which enhances the overall notification experience.
• Background and Terminated State Notifications
In the background, or terminated state, notifications are automatically handled by the system and appear in the notification tray. You can use Firebase’s built-in FCM message handling for this, so no additional code is needed for these states.
Configuring Notification Channels (Android)
On Android, notifications are delivered through channels. You can configure channels with custom sounds, vibration patterns, and importance levels.
Before displaying a notification in Notifee, set up a channel:import notifee from ‘@notifee/react-native’;
async function createNotificationChannel() {
await notifee.createChannel({
id: ‘default’,
name: ‘Default Channel’,
importance: AndroidImportance.HIGH,
});
}
useEffect(() => {
createNotificationChannel();
}, []);
Testing Push Notifications
1. Sending a Test Notification
You can send test notifications from the Firebase Console by navigating to the Cloud Messaging section and sending a message to a specific device using the FCM token.
2. Handling Notification Clicks
Notifee also allows you to handle notification interaction (e.g., when the user taps on the notification).notifee.onBackgroundEvent(async ({ type, detail }) => {
if (type === notifee.EventType.ACTION_PRESS) {
console.log(‘Notification tapped: ‘, detail.notification);
}
});
Resources:
• React Native Firebase Documentation
Author: Mahir Uslu